May 24, 2014
Technology问题
在txt里面打印1-10里面随机的9个数。
思路
如何生成随机数?
Google “generate random python” , 结果如下:
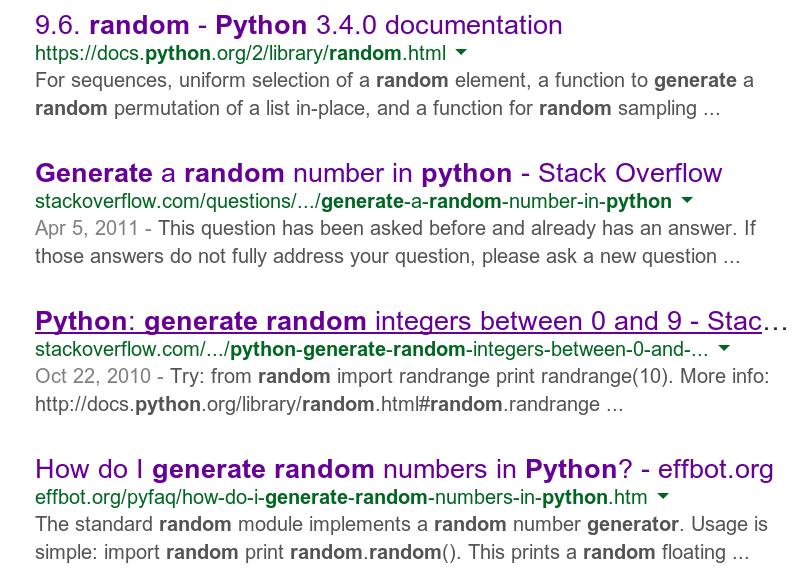
挨个看,马上你会发现下面这个网页有答案:
http://stackoverflow.com/questions/5555712/generate-a-random-number-in-python
启动终端试验之:
$ python
Python 2.7.6 (default, Feb 26 2014, 12:07:17)
[GCC 4.8.2 20140206 (prerelease)] on linux2
Type "help", "copyright", "credits" or "license" for more information.
>>> from random import randint
>>> randint(2,9)
3
>>> randint(1,10)
9
>>> randint(1,10)
4
如何循环打印
照样Google, 搜索关键字” python loop times”,结果如下:
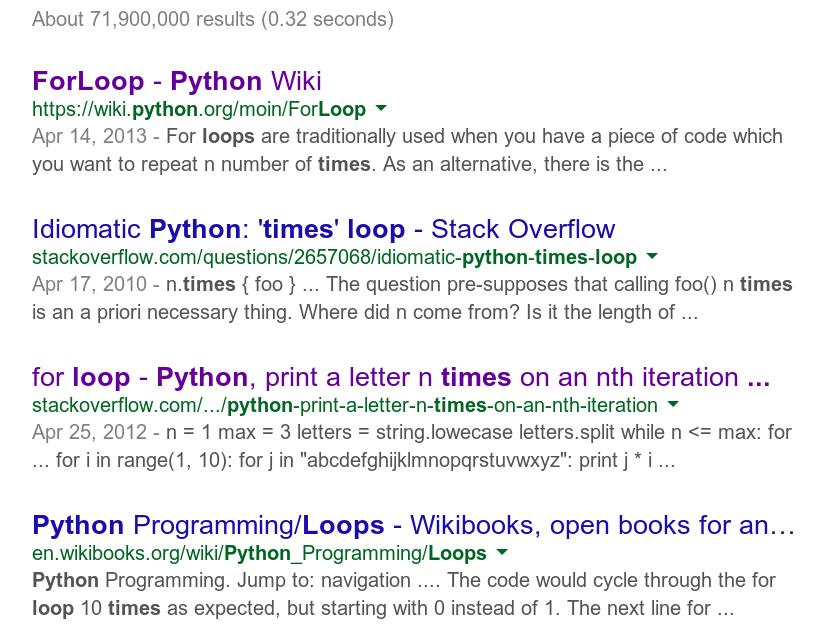
随便点点看, 发现python自己的文档里就已经有很详细的关于loop的例子了:
https://wiki.python.org/moin/ForLoop
试验之:
>>> for x in range(0,3):
... print "hello"
...
hello
hello
hello
综合,得出答案
用for的实现:
>>> for x in range(0,9):
... print random.randrange(1,10)
...
2
5
3
7
9
4
7
3
1
发散到用while的实现(原代码见上面的wiki网页)
>>> x = 1
>>> while x < 10:
... print random.randrange(1,10)
... x += 1
...
4
4
4
5
1
9
1
8
2
当然你还可以反着弄while:
>>> x = 10
>>> while x > 0:
等等等等,不提示了
May 24, 2014
TechnologyFont Customization
Install following fonts:
$ sudo pacman -S wqy-bitmapfont wqy-zenhei ttf-arphic-ukai ttf-arphic-uming ttf-fireflysung
And then for more beautiful font, refer to:
http://kkkttt.github.io/blog/2013/12/25/archlinuxzhong-wen-hua-wen-ti/
VIM Customization
Vim is my favorite editor, so maintain a configuration file is necessary for deploying it on various machines.
$ sudo pacman -S ctags
Then copy the default vimrc file from /usr/share/vim74/vimrc_example to your own directory, named it into .vimrc.
Add folllowing lines into .vimrc file:
" Customization Start
set nu
set cursorline
set cursorcolumn
"Enable syntax
syntax enable
syntax on
" colorscheme solarized
autocmd BufNewFile,BufReadPost *.ino,*.pde set filetype=cpp
"进行Tlist的设置
""TlistUpdate可以更新tags
map <F3> :silent! Tlist<CR>
"按下F3就可以呼出了
let Tlist_Ctags_Cmd='ctags' "因为我们放在环境变量里,所以可以直接执行
let Tlist_Use_Right_Window=1 "让窗口显示在右边,0的话就是显示在左边
let Tlist_Show_One_File=0 "让taglist可以同时展示多个文件的函数列表,如果想只有1个,设置为1
let Tlist_File_Fold_Auto_Close=1 "非当前文件,函数列表折叠隐藏
let Tlist_Exit_OnlyWindow=1 "当taglist是最后一个分割窗口时,自动推出vim
let Tlist_Process_File_Always=0 "是否一直处理tags.1:处理;0:不处理。不是一直实时更新tags,因为没有必要
let Tlist_Inc_Winwidth=0
set fileencodings=utf8,cp936,gb18030,big5
"In visual mode, you can simply press ,x and Vim will filter your content
"through tidy.
:vmap ,x :%!tidy -q -i --show-errors 0<CR> "Auto command for clear the html files
:command Thtml :%!tidy -q -i --show-errors 0
:command Txml :%!tidy -q -i --show-errors 0 -xml
" Setting up Vundle - the vim plugin bundler
let iCanHazVundle=1
let vundle_readme=expand('~/.vim/bundle/vundle/README.md')
if !filereadable(vundle_readme)
echo "Installing Vundle.."
echo ""
silent !mkdir -p ~/.vim/bundle
silent !git clone https://github.com/gmarik/vundle ~/.vim/bundle/vundle
let iCanHazVundle=0
endif
set rtp+=~/.vim/bundle/vundle/
call vundle#rc()
Bundle 'gmarik/vundle'
Bundle 'tpope/vim-fugitive'
Bundle 'taglist.vim'
" Bundle 'molokai'
"Add your bundles here
"Bundle 'Syntastic' "uber awesome syntax and errors highlighter
Bundle 'altercation/vim-colors-solarized'
Bundle 'https://github.com/tomasr/molokai.git'
"T-H-E colorscheme
"Bundle 'https://github.com/tpope/vim-fugitive' "So awesome, it should be illegal
"...All your other bundles...
if iCanHazVundle == 0
echo "Installing Bundles, please ignore key map error messages"
echo ""
:BundleInstall
endif
" Setting up Vundle - the vim plugin bundler end
colorscheme molokai
set guifont=YaHei\ Consolas\ Hybrid\ 11.5
Then everything I want is OK.
Command for configurating vbundle:
BundleInstall
BundleSearch
Tree tool has been installed, for view the complex directory structure :
$ sudo pacman -S tree
Install arduino from yaourt.
$ yaourt -S arduino
This will also install avr-gcc.
NodeJS
Install via yaourt:
$ yaourt -S nodejs
Basic Tutorial:
http://localhost2/blog/2014/05/14/node-dot-js-quick-start/
Later I will add the “Chat-like” Terminal-emulator series, so this part could be enhanced.
Tools which used for writing articles.
$ sudo pacman -S blender dia inkscape scribus
$ yaourt yed
$ yaourt sublime
$ sudo pacman -S gedit
$ sudo pacman -S imagemagick
Awesome Configuration
Dmenu which used for run applications:
$ sudo pacman -S dmenu
Screen shot:
$ sudo pacman -S scrot
Edit the /usr/bin/mycapscr
[Trusty@~]$ cat /usr/bin/mycapscr
scrot -s '%Y_%m_%d_%H_%M_%S_$wx$h.jpg' -e 'mv $f ~/capscr/'
Tcpdump for capturing packets.
$ sudo pacman -S tcpdump
Whatpulse for recording key click times:
$ sudo yaourt -S whatpulse
$ sudo gpasswd -a Trusty input
Remote Desktop:
$ pacman -S rdesktop
Conky and Conky configuration file.
$ pacman -S conky
hex tool:
$ pacman -S ghex
Calculator:
$ pacman -S bc
$ pacman -S gnome-calculator
Nautlus:
$ pacman -S nautilus
rar:
$ pacman -S rar
autossh:
$ pacman -S autossh
cmake:
$ pacman -S cmake
cronie:
$ pacman -S connie
elinks:
$ pacman -S elinks
eog:
$ pacman -S eog
ethtool
$ pacman -S ethtool
expect:
$ pacman -S expect
feh:
$ pacman -S feh
gparted:
$ pacman -S gparted
ipython2:
$ pacman -S ipython2
ntfs-3g:
$ pacman -S ntfs-3g
sakura lilyterm:
$ pacman -S sakura lilyterm
tmux:
$ pacman -S tmux
w3m,lynx:
$ pacman -S w3m lynx
xclip:
$ pacman -S xclip
xlockmore:
$ pacman -S xlockmore
XPdf:
$ pacman -S xpdf
calibre:
$ pacman -S calibre
Rstudio:
$ yaourt rstudio-desktop-bin
pandoc(Problem!):
$ yaourt pandoc
# Notice, you have to add curl -k in /etc/makepkg.conf
briss(For cutting pdf into 6 inch):
$ yaourt briss
coolreader(For reading epub):
$ yaourt -S coolreader
May 23, 2014
TechnologyWriting Blog
I use octopress for writing blog, so this time in USB system I also want to enable it.
Edit the /etc/hostname
and name my computer into “USBArch”, because we want to setup the id_rsa.pub in next step.
$ ssh-keygen
$ cat ~/.ssh/id_rsa.pub
Copy the content and add it into the “https://github.com/settings/ssh”,
Then use command ssh -T git@github.com
to verify if you can successfully be authenticated.
$ git clone git@github.com:kkkttt/debian_octopress.git
$ git clone git@github.com:kkkttt/herokublog.git
Now the repository is OK, but the ruby environment should be set.
$ curl -L https://get.rvm.io | bash -s stable
$ rvm use 1.9.3
# log out the terminal and log in again
$ rvm rubygems latest
$ cdheroku
# Choose yes, trust the .rvmrc
$ gem install bundler
$ bundle install
Test the rake command:
$ rake generate && rake deploy
OK, Then we can octopress for writing blogs.
WebServer
We will use xampp for webserver, because it’s very convinient to configure. Login as root, then:
# yaourt xampp
The detailed configuration steps could be viewed at:
http://kkkttt.github.io/blog/2014/04/23/deploy-xampp-on-archlinux/
In fact here I met a very strange problem, it seems if I put the whole blog under /home/Trusty/code
, then the browser will complains that 403, so here I make a trick in rakefile(Which is used for generating the static website), add following line in rake generate
desc "Generate jekyll site"
task :generate do
raise "### You haven't set anything up yet. First run `rake install` to set up an Octopress theme." unless File.directory?(source_dir)
puts "## Generating Site with Jekyll"
system "compass compile --css-dir #{source_dir}/stylesheets"
system "jekyll"
system "sudo cp -ar ./public/ /opt/ && sudo chown -R nobody /opt/public/ && sudo chgrp -R nobody /opt/public/ && sudo chmod 755 -R /opt/public/"
end
I don’t find another method, because xampp is too complex for configuring, we needn’t spend much more time on it.
Tomorrow I will setup the other language development environment.
May 22, 2014
TechnologyTrouble Shooting
Unfortuately the qemu based pysical disk can’t bootup the machine correctly, so I re-intall the sytem on USB-Disk from the scratch. This time the problem appears as another: It can startup the machine, but failed to boot-up in qemu.
So I have to changed to use VirtualBox for booting the system.
Get the disk id via:
$ ls -l /dev/disk/by-id
lrwxrwxrwx 1 root root 9 May 22 14:45 usb-ATA_ST980811AS_xxxxxxxx-0:0 -> ../../sdb
Then use VirtualBox’s Internal command for creating the vmdk file, this vmdk file actually contains the physical disk.
$ VBoxManage internalcommands createrawvmdk -filename ./rawusb1.vmdk -rawdisk /dev/disk/by-id/usb-ATA_ST980811AS_XXXXXXXXXXX-0:0
RAW host disk access VMDK file ./rawusb1.vmdk created successfully.
$ sudo chown -R Trusty *
Now create a new virtual machine in virtualbox, use the newly created rawusb1.vmdk, bootup and you got the physical disk based virtual machine running.
Continue Install System
Install virtualenvironment via:
sudo pacman -S python2-virtualenv python-virtualenv python-virtualenvwrapper
In company machine, use pip freeze for getting all of the packages:
$ workon venv2
$ pip freeze>requirement.txt
In usb-disk based machine, install from the requirement.txt
$ mkdir .virtualenvs
# Add following lines into the .bashrc
export WORKON_HOME=~/.virtualenvs
source /usr/bin/virtualenvwrapper.sh
$ mkvirtualenv -p /usr/bin/python2.7 venv
$ pip install -r requirements.txt
After we update the installation, the package is the same as in computer machine.
ZSH
Install zsh and use it for replacing default bash:
$ sudo pacman -S zsh
$ chsh -s $(which zsh)
Now we can download the .zshrc from the company machine to usb disk based system.
Setting the proxy and set it for git using proxy:
(venv)[Trusty@localhost ~]$ gcc -o connect connect.c
(venv)[Trusty@localhost ~]$ sudo mv connect /usr/bin/
(venv)[Trusty@localhost ~]$ sudo chmod 777 /usr/bin/connect
(venv)[Trusty@localhost ~]$ sudo vim /usr/bin/myproxy
(venv)[Trusty@localhost ~]$ sudo chmod 777 /usr/bin/myproxy
(venv)[Trusty@localhost ~]$ cat /usr/bin/myproxy
/usr/bin/connect -H 1xx.xxx.xxx.xxx:2xxxx $@
(venv)[Trusty@localhost ~]$ git config --global core.gitproxy "/usr/bin/myproxy for *.*"
(venv)[Trusty@localhost ~]$ git config --global user.name "feixxxx"
(venv)[Trusty@localhost ~]$ git config --global user.email "feixxxx@gmail.com"
BlueTooth
Install blueman:
$ sudo pacman -S patch automake autoconf libtool
$ yaourt blueman
# choose blueman-bzr
$ sudo pacman -S bluez-utils bluez-libs python2-pybluez
$ yaourt -S bluez4
$ yaourt pulseaudio-bluez4
Configure bluetooth:
$ sudo systemctl start bluetooth
$ sudo systemctl enable bluetooth
$ cat /etc/bluetooth/audio.conf
[General]
Enable=Socket
[A2DP]
SBCSources=1
$
Later bluemanager will be added into the awesome startup application.
The configuration is pretty complex, this is the start-point for settingup the bluetooth, later we will configure the bluetooth headset.
Tray items
$ sudo pacman -S udiskie wicd wicd-gtk
Awesome Customization
Download the themes from github:
$ sudo pacman -S hddtemp vicious
$ git clone https://github.com/Morley93/awesome-themes-3.5.git
Still have some problems. Need update.
Then get the customized awesome configuration file. Be sure to use the default, because later you can customize yourself.
Solve wicd error:
Error: Could not connect to wicd’s D-Bus interface
$ sudo pacman -R wicd wicd-gtk
$ sudo rm -rf /etc/wicd /var/log/wicd /etc/dbus-1/system.d/wicd*
$ sudo pacman -S wicd wicd-gtk
$ sudo pacman -Syu systemd-sysvcompat
$ sudo gpasswd -a Trusty users
$ sudo systemctl enable wicd
$ sudo systemctl start wicd
Now your wicd is OK for see.
udiskie2 problem, cannot start because lack of PyYAML:
$ sudo pacman -S python2-pip
$ sudo pip2 install PyYAML
Now seems all of your tray icons will be OK.
Install the mlocate so we can use updatedb:
$ sudo pacman -S mlocate
$ sudo updatedb
May 21, 2014
TechnologyBackground
Since I want to hava a usb-based OS which could easily be taken by hand, I took this series for resarching how to finish this aim.
Preparation
ArchLinux iso file, USB-3.0 HardDisk, 80GB, later I will use a bigger one.
Qemu Script
I use qemu firstly to install the system. following is the configuraton file for qemu-i386:
#!/bin/bash
USERID=$(whoami)
# Get name of newly created TAP device; see https://bbs.archlinux.org/viewtopic.php?pid=1285079#p1285079
precreationg=$(/usr/bin/ip tuntap list | /usr/bin/cut -d: -f1 | /usr/bin/sort)
sudo /usr/bin/ip tuntap add user $USERID mode tap
postcreation=$(/usr/bin/ip tuntap list | /usr/bin/cut -d: -f1 | /usr/bin/sort)
IFACE=$(comm -13 <(echo "$precreationg") <(echo "$postcreation"))
# This line creates a random MAC address. The downside is the DHCP server will assign a different IP address each time
printf -v macaddr "52:54:%02x:%02x:%02x:%02x" $(( $RANDOM & 0xff)) $(( $RANDOM & 0xff )) $(( $RANDOM & 0xff)) $(( $RANDOM & 0xff ))
# Instead, uncomment and edit this line to set a static MAC address. The benefit is that the DHCP server will assign the same IP address.
# macaddr='52:54:be:36:42:a9'
qemu-system-i386 -net nic,macaddr=$macaddr -net tap,ifname="$IFACE" $*
sudo ip link set dev $IFACE down &> /dev/null
sudo ip tuntap del $IFACE mode tap &> /dev/null
Run the virtual machine like following:
$ ./run-qemu-i386 -hda /dev/sdc -m 1024 -boot d -cdrom ./archlinux-2014.05.01
Installation Of ArchLinux
When your iso bootup, you will enter command-line, use following command for start the ssh :
# passwd root
# systemctl start sshd
# ifconfig
# ssh root@10.0.0.240
Partition disk:
# fdisk /dev/sda
Command (m for help): p
Disk /dev/sda: 74.5 GiB, 80026361856 bytes, 156301488 sectors
Units: sectors of 1 * 512 = 512 bytes
Sector size (logical/physical): 512 bytes / 512 bytes
I/O size (minimum/optimal): 512 bytes / 512 bytes
Disklabel type: dos
Disk identifier: 0xc001c001
Device Boot Start End Blocks Id System
/dev/sda1 2048 156301487 78149720 83 Linux
Command (m for help): d
Selected partition 1
Partition 1 has been deleted.
Command (m for help): n
Partition type:
p primary (0 primary, 0 extended, 4 free)
e extended
Select (default p): p
Partition number (1-4, default 1): 1
First sector (2048-156301487, default 2048):
Last sector, +sectors or +size{K,M,G,T,P} (2048-156301487, default 156301487): +1G
Created a new partition 1 of type 'Linux' and of size 1 GiB.
Command (m for help): t
Hex code (type L to list all codes): 82
Changed type of partition 'Linux' to 'Linux swap / Solaris'.
Command (m for help): n
Partition type:
p primary (1 primary, 0 extended, 3 free)
e extended
Select (default p): p
Partition number (2-4, default 2):
First sector (2099200-156301487, default 2099200):
Last sector, +sectors or +size{K,M,G,T,P} (2099200-156301487, default 156301487):
Created a new partition 2 of type 'Linux' and of size 73.5 GiB.
Command (m for help): w
The partition table has been altered.
Calling ioctl() to re-read partition table.
Syncing disks.
root@archiso ~ # fdisk -l /dev/sda
Disk /dev/sda: 74.5 GiB, 80026361856 bytes, 156301488 sectors
Units: sectors of 1 * 512 = 512 bytes
Sector size (logical/physical): 512 bytes / 512 bytes
I/O size (minimum/optimal): 512 bytes / 512 bytes
Disklabel type: dos
Disk identifier: 0xc001c001
Device Boot Start End Blocks Id System
/dev/sda1 2048 2099199 1048576 82 Linux swap / Solaris
/dev/sda2 2099200 156301487 77101144 83 Linux
Now your partition is ok, make filesystems.
# mkfs.ext4 /dev/sda2
root@archiso ~ # mkswap /dev/sda1
root@archiso ~ # swapon /dev/sda1
Now begin to install system:
# mount /dev/sda2 /mnt
# pacstrap /mnt base
Configuraion:
# genfstab -p /mnt >> /mnt/etc/fstab
# arch-chroot /mnt
sh-4.3# cat /etc/hostname
FreeArch
sh-4.3# ln -s /usr/share/zoneinfo/Asia/Shanghai /etc/localtime
# Uncomment the items in /etc/locale.gen, and run:
sh-4.3# locale-gen
Generating locales...
en_US.UTF-8... done
en_US.ISO-8859-1... done
zh_CN.GB18030... done
zh_CN.GBK... done
zh_CN.UTF-8... done
zh_CN.GB2312... done
zh_TW.EUC-TW... done
zh_TW.UTF-8... done
zh_TW.BIG5... done
Generation complete.
sh-4.3# mkinitcpio -p linux
sh-4.3# passwd root
Network Configuration:
sh-4.3# ip link
1: lo: <LOOPBACK,UP,LOWER_UP> mtu 65536 qdisc noqueue state UNKNOWN mode DEFAULT group default
link/loopback 00:00:00:00:00:00 brd 00:00:00:00:00:00
2: ens3: <BROADCAST,MULTICAST,UP,LOWER_UP> mtu 1500 qdisc pfifo_fast state UP mode DEFAULT group default qlen 1000
link/ether 52:54:94:b5:12:24 brd ff:ff:ff:ff:ff:ff
sh-4.3# pacman -S dhcpcd
sh-4.3# systemctl enable dhcpcd@ens3
ln -s '/usr/lib/systemd/system/dhcpcd@.service' '/etc/systemd/system/multi-user.target.wants/dhcpcd@ens3.service'
BootLoad Configuration:
# pacman -S grub
# grub-install --target=i386-pc --recheck --debug /dev/sda
# grub-mkconfig -o /boot/grub/grub.cfg
Umount and reboot:
umount -R /mnt
Configuration Of System
Install openssh firstly, and net-tools for getting the ipaddress using ifconfig:
pacman -S openssh
systemctl enable sshd
systemctl start sshd
pacman -S net-tools
Now you can use terminal for getting to the qemu-based machine, and sshd service should be enabled always.
Quickly setup an account, because using root is a bad idea.
# useradd -m -g root -G audio -s /bin/bash Trusty
# pacman -S sudo
# visudo
##
## User privilege specification
##
root ALL=(ALL) ALL
Trusty ALL=(ALL) NOPASSWD: ALL
## Locale settings
# Defaults env_keep += "LANG LANGUAGE LINGUAS LC_* _XKB_CHARSET"
Defaults env_keep += "http_proxy https_proxy ftp_proxy ftps_proxy"
Now you can use the newly added username “Trusty” for login.
Install yaourt, add following lines into the file /etc/pacman.conf:
[archlinuxfr]
SigLevel = Never
Server = http://repo.archlinux.fr/$arch
Then update and install yaourt:
sudo pacman -Syu && sudo pacman -S yaourt
Next chapter we will begin to install softwares.